Nettyfy Technologies has a expert team of Angular Developers and always ready to share a their knowledge with others. In this article, we are explain about promises and observable in Angular JavaScript. ES6 Promises and RxJS Observables are both used to handle async activity in JavaScript. While an Observable can do everything a Promise can, the reverse is not true.
What is a Promise?
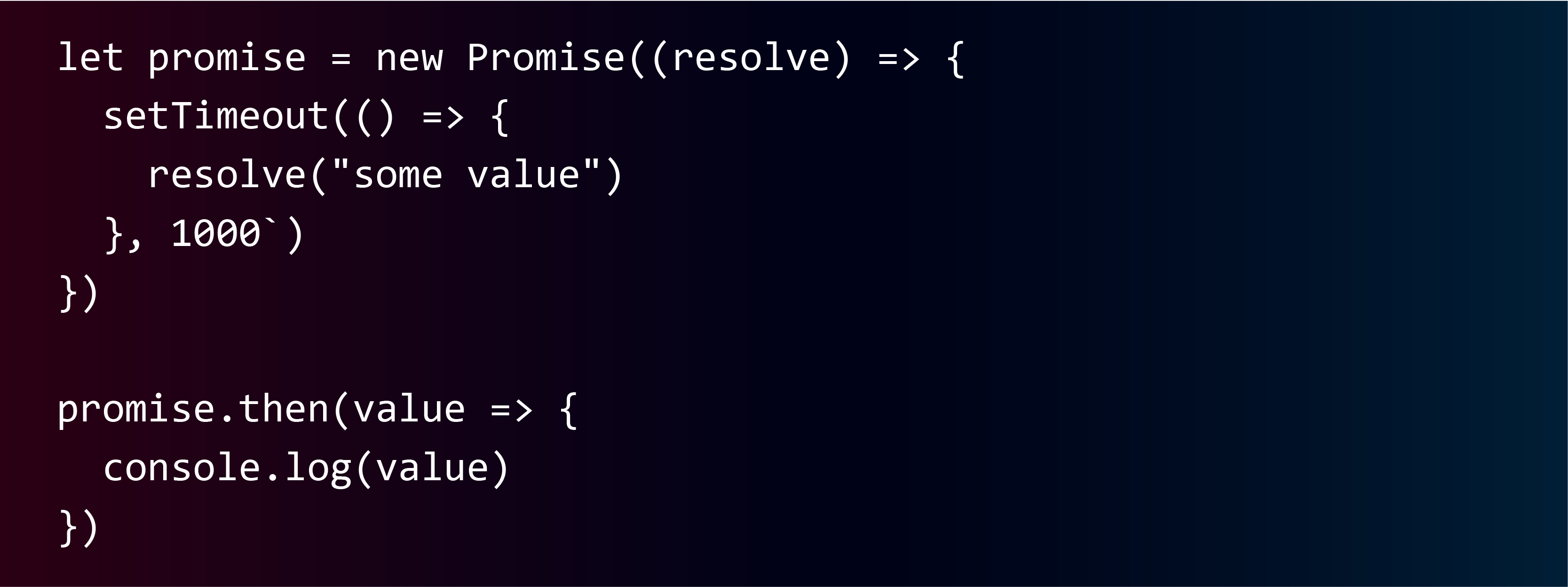
A Promise is a more elegant way of handling async activity in JavaScript. Promises in Angular provide an easy way to execute asynchronous functions that use callbacks, while emitting and completing (resolving or rejecting) one value at a time.
Example of a Promise:
let promise = new Promise((resolve) => {
setTimeout(() => {
resolve(“some value”)
}, 1000)
})
promise.then(value => {
console.log(value)
})
Output:
some value
A Promise works by taking a function with (optionally) two arguments resolve and reject. The passed in function executes some async code and either resolves or rejects.
Once the Promise resolves, its then() method executes a handler function with the emitted value.
What is Observable?
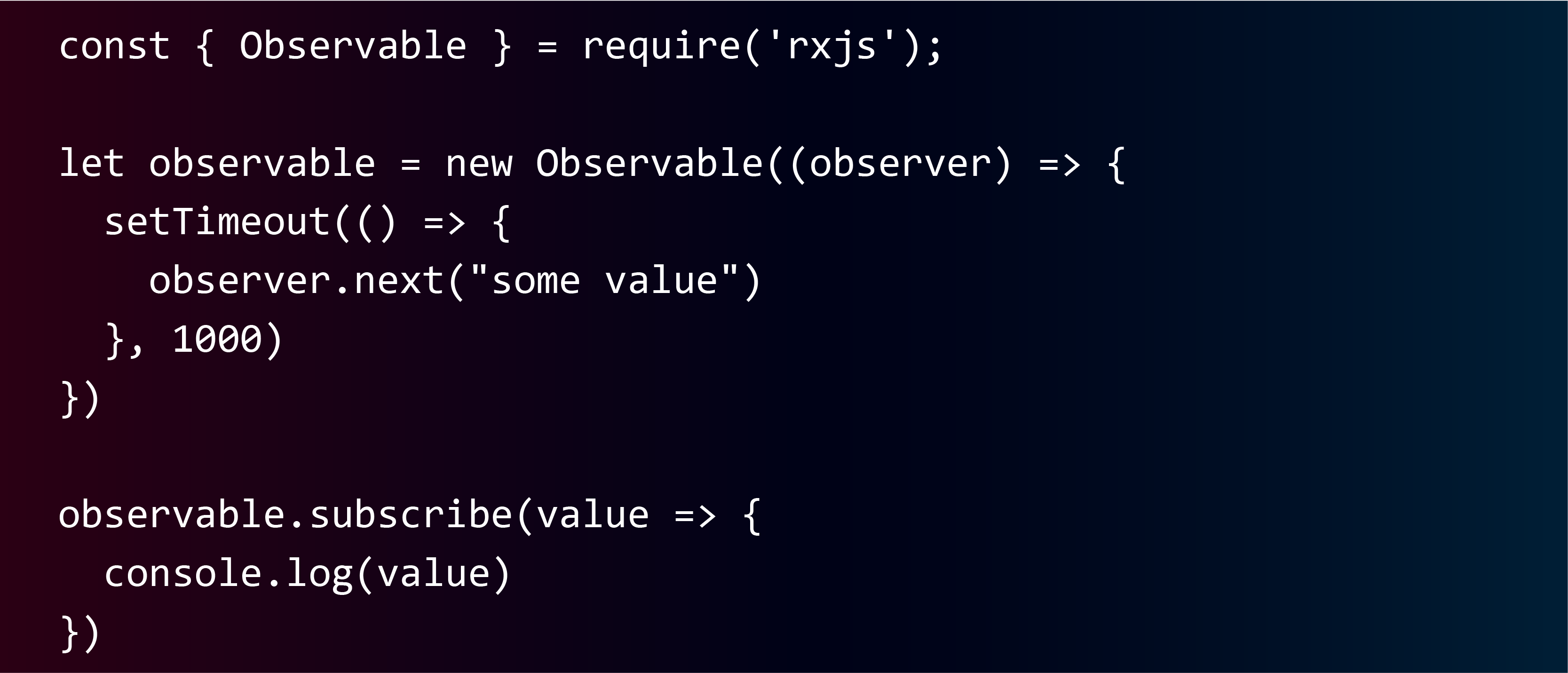
Observables are the RxJS implementation of the observer design pattern. Observables are data source wrappers and then the observer executes some instructions when there is a new value or a change in data values.
Observables are an alternative to Promises for handling async activity:
Example of Observable:
const { Observable } = require(‘rxjs’);
let observable = new Observable((observer) => {
setTimeout(() => {
observer.next(“some value”)
}, 1000)
})
observable.subscribe(value => {
console.log(value)
})
Output:
some value
Unlike the Promise, Observables aren’t native to JavaScript. This requires us to import the RxJS library:
const { Observable } = require(‘rxjs’);
While you can implement the observer design pattern yourself, the “Observable” in JavaScript usually refers to the RxJS implementation.
For more information on Observables in JavaScript, check out JavaScript Observables.
Differences Between Observables and Promises:
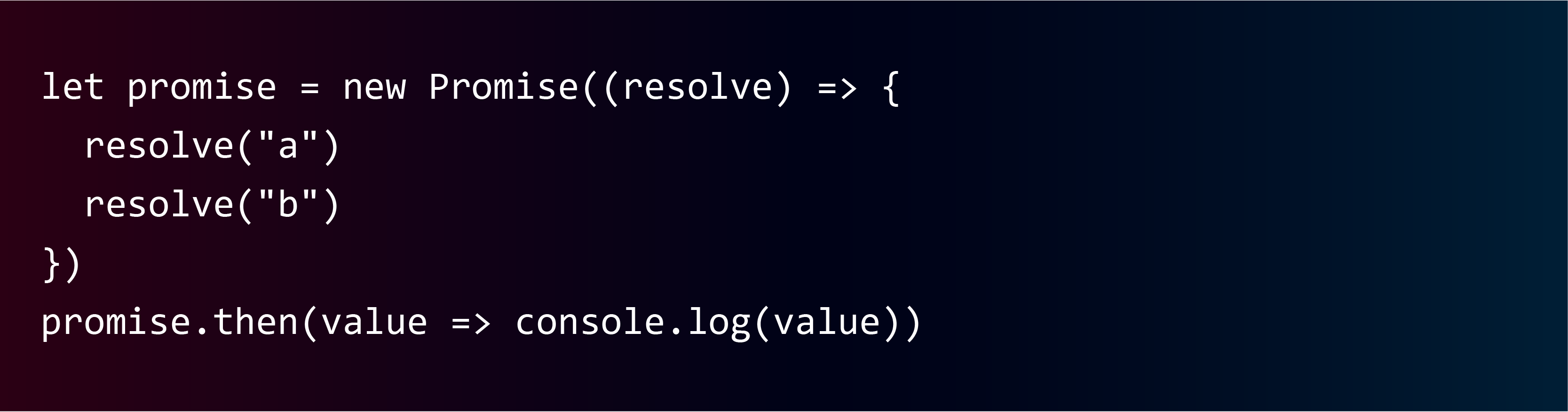
A Promise emits a single value:
let promise = new Promise((resolve) => {
resolve(“a”)
resolve(“b”)
})
promise.then(value => console.log(value))
Output: a
An Observable can emit multiple values:
let observable = new Observable((observer) => {
observer.next(“a”)
observer.next(“b”)
})
observable.subscribe(value => {
console.log(value)
})
Output: a b
Once a Promise resolves, that’s it. You can’t call resolve twice and get different values. Only the first value a is returned by the promise.
An Observable can emit multiple values. Calling next() executes the handler function each time. Both a and b are returned.
Here are some key points:
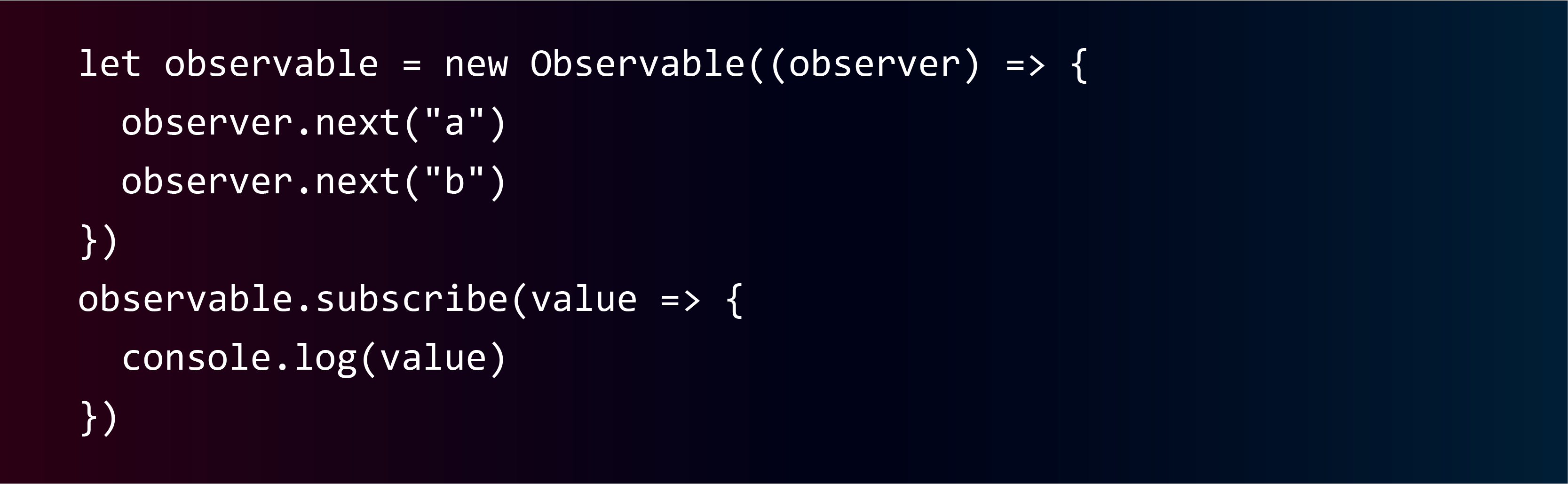
1) Definition: “Observable and Observer” is a pattern of message passing from publisher to subscriber.
2) Flow of functionality:
● Observable is created
● Observer subscribe to Observable
● Observable can pass message to observer
● each time, when the observable passes not a message it is received by Observer
3) Real-time usage of Observable and Observer
● While receiving response from AJAX
● While performing large tasks in client(browser)
Observer : Any object that wishes to be notified when the state of another object changes.
Observable : Any object whose state may be of interest, and in whom another object may register an interest.