In this article, we will explore what middleware is in Redux, why it’s used, and how you can create your own middleware from scratch. As a leading JavaScript development company in USA and India, you can go through the important points of this new version.
So let’s get started.
What Is Redux Middleware?
Redux Middlewares are simple functions which allow us to intercept every action which is sent to the reducer and we can modify or cancel the action.
Take a look at the below code:
// code snippet
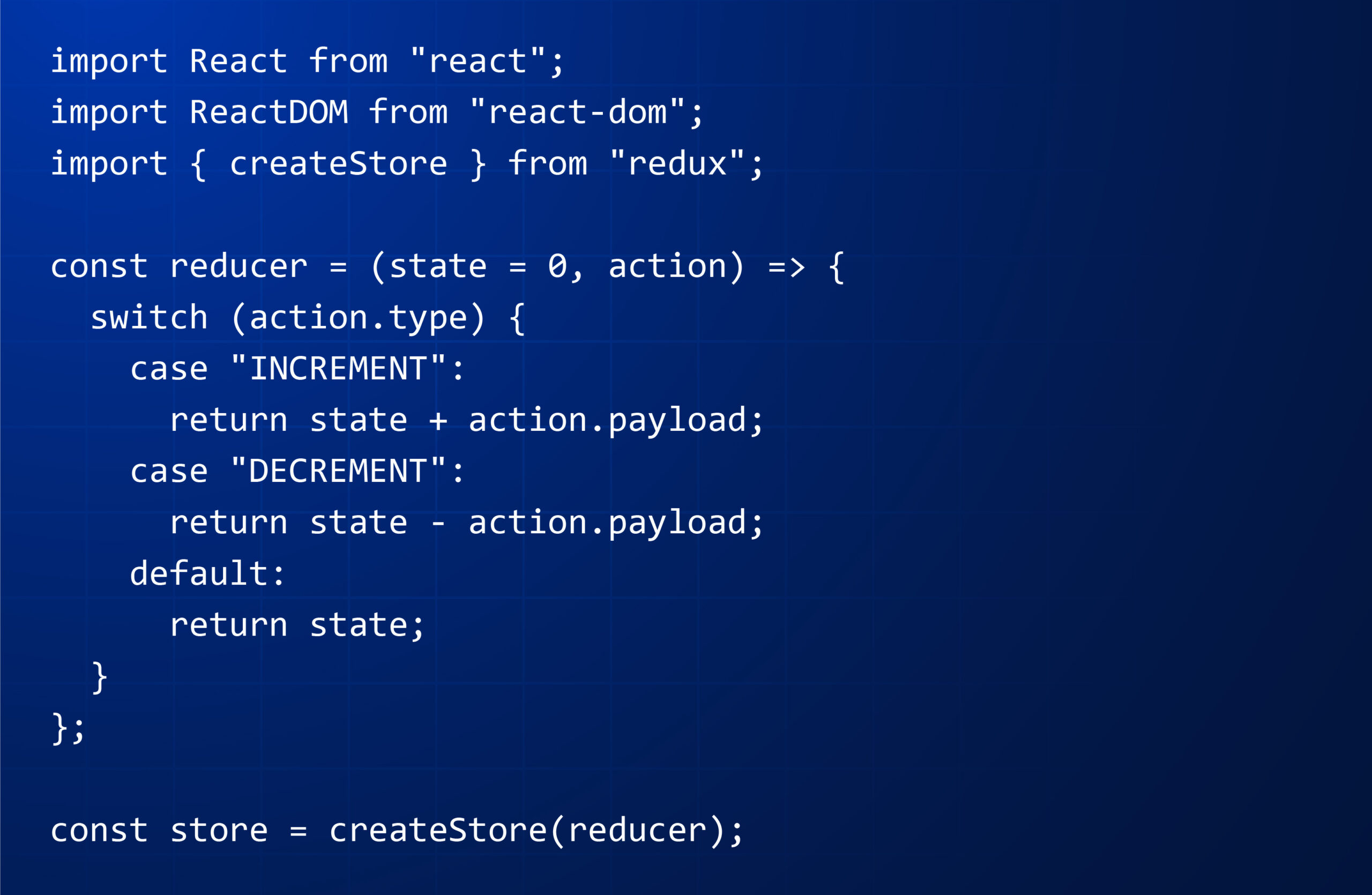
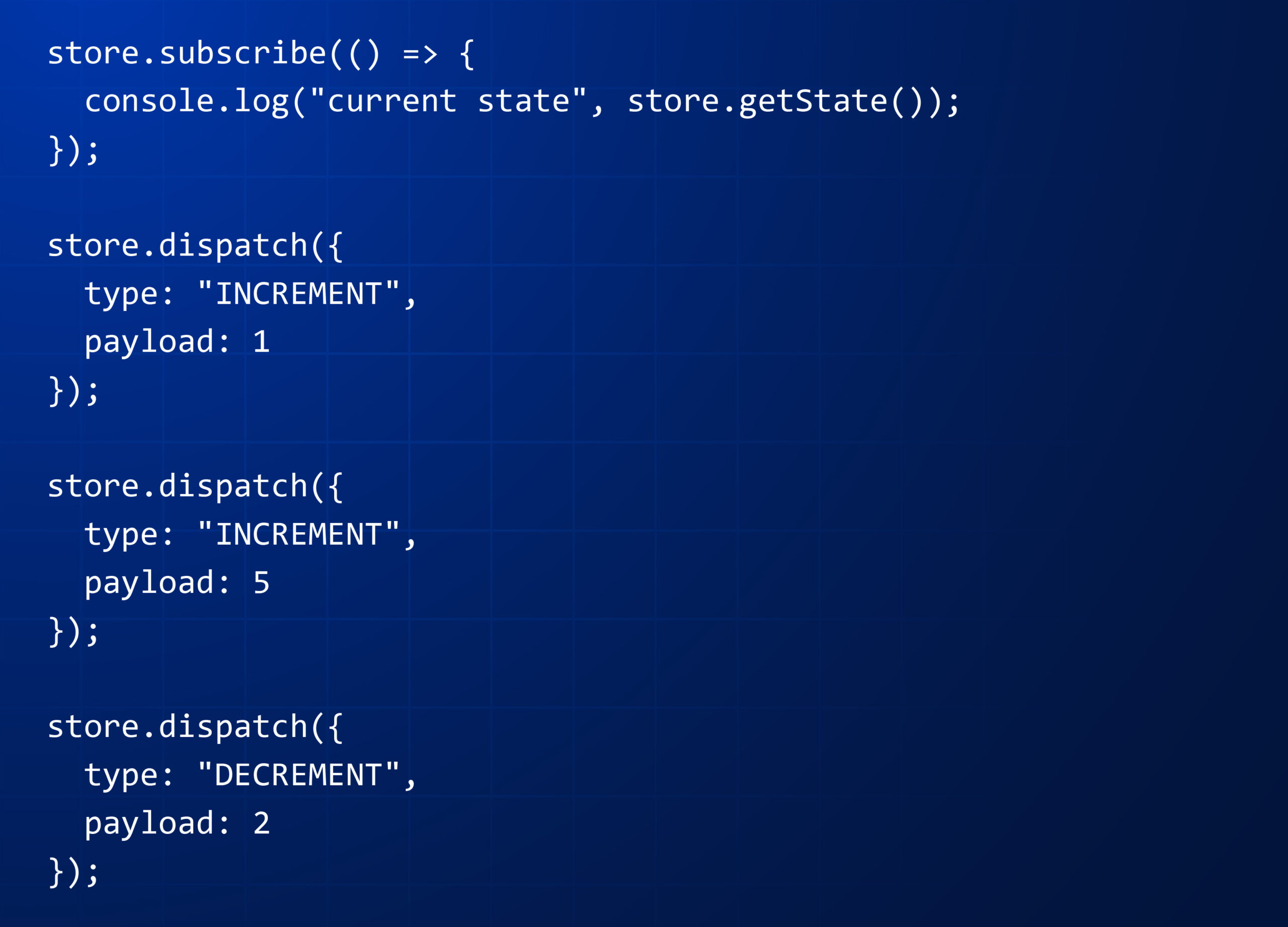
Explanation:
1. First import the createStore function from the redux package.

2. The CreateStore function accepts 3 arguments:
● The first one is the reducer, a function which is required.
● Second one is a state , initial value of which is optional.
● Third one is a middleware, a kind of function, this argument is also optional.
How to Create Middleware in React
In order to create a middleware, we will use applyMiddleware, which is present in the redux package. So, first import the applyMiddleware function.
// code snippet

Let’s say we are creating a loggerMiddleware. You need to use the following syntax:
// code snippet

The above code is similar to the code given below:
// code snippet
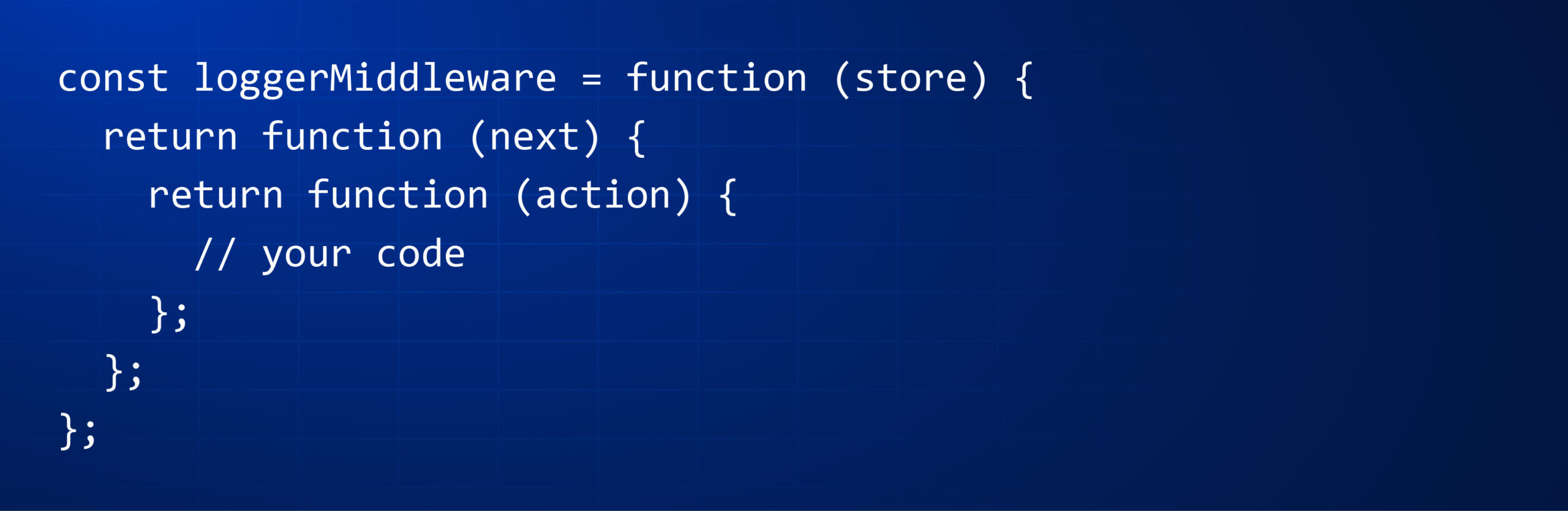
Now we have created our middleware function , we pass it to the applyMiddleware function like this :
// code snippet

And finally, we pass the above middleware to the createStore function like this:
// code snippet

You might be surprised to see this. Initially, we discussed that the createStore function accepts 3 arguments; middleware is the 3rd argument. And we are providing the middleware function as a 2 argument. The second argument (initial state) is optional. Based on the type of arguments, the CreateStore function will automatically identify that the passed argument is a middleware because it has the specific syntax of nested functions.
The middleware function will look like this:
// code snippet
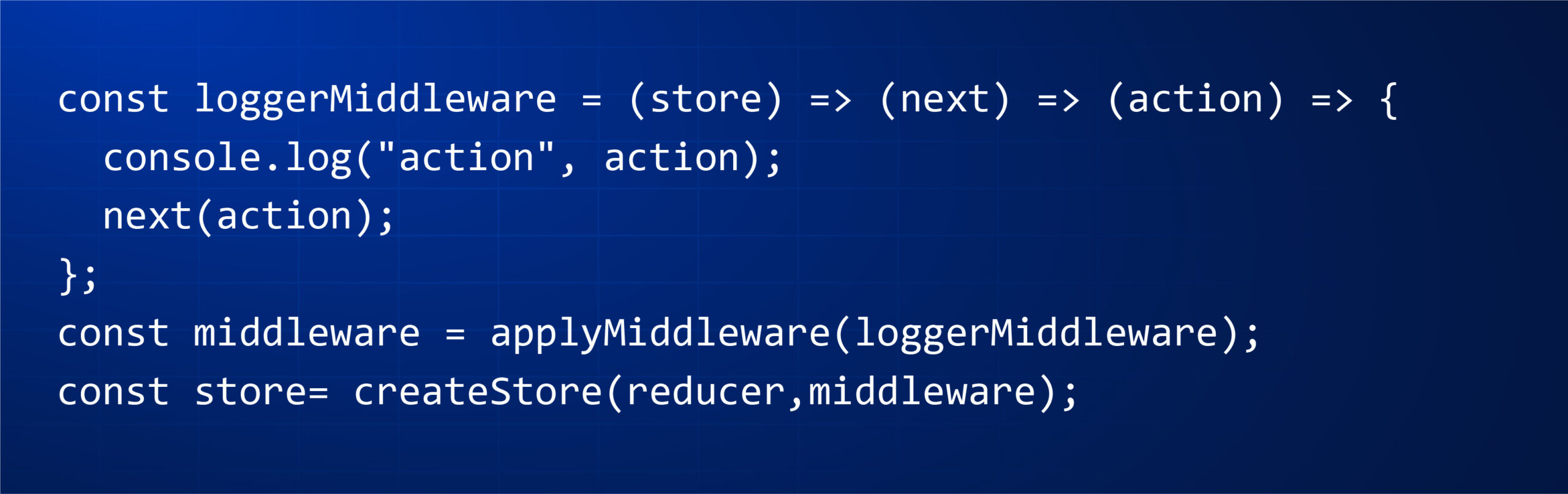
If you check the console, you will see the following output:
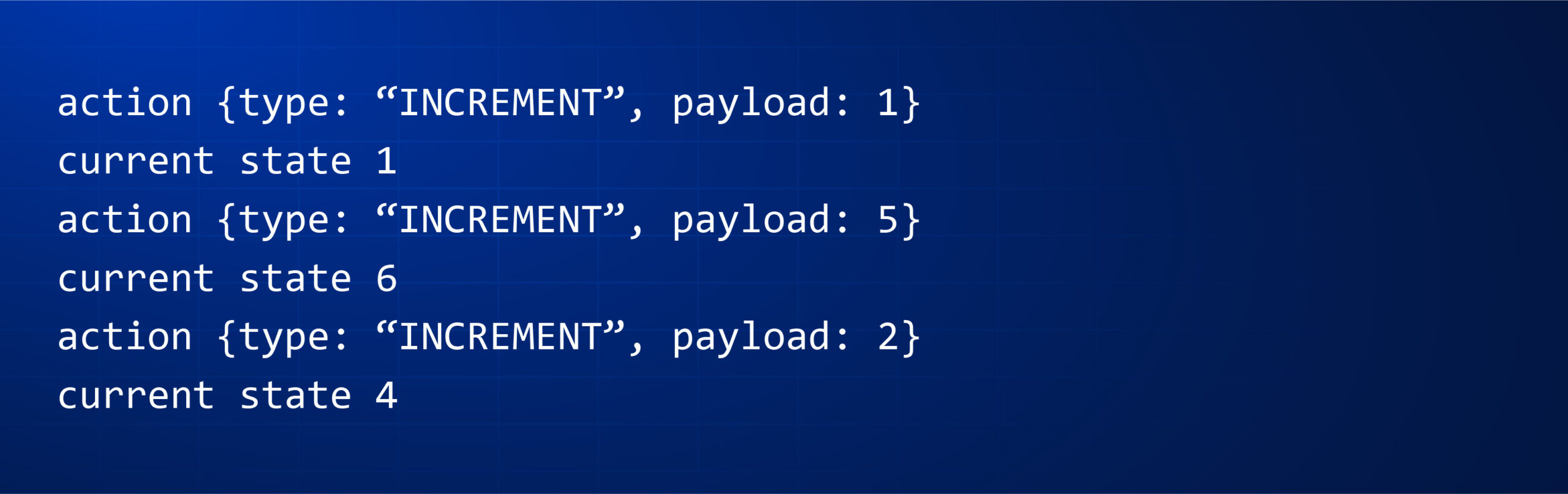
Before the action is dispatched to the store, the middleware gets executed, as we can see the action logged to the console.
Now, what will happen if we don’t call the next function inside the loggerMiddleware ?
Then the action will not be sent to the reducer, so the store will not be updated.
If you update your function like this
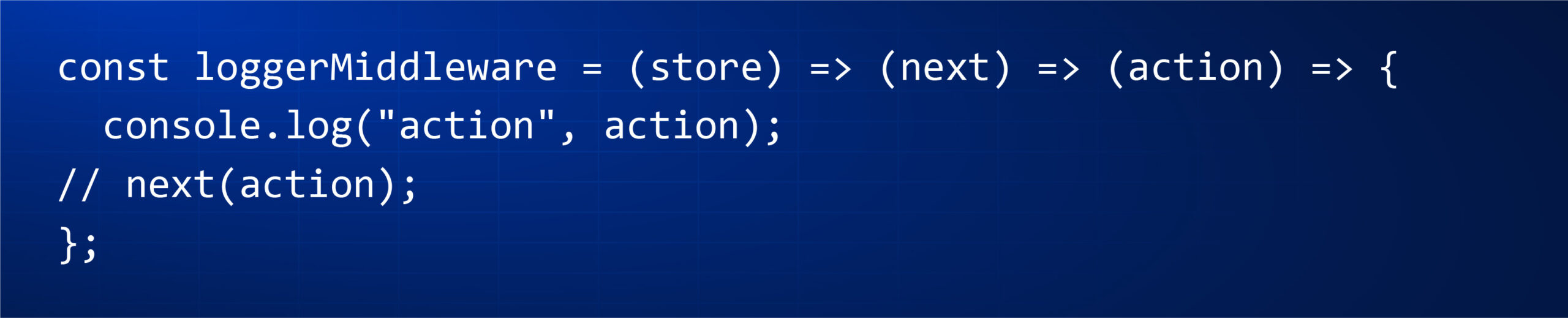
If you check the console, you will see the following output :
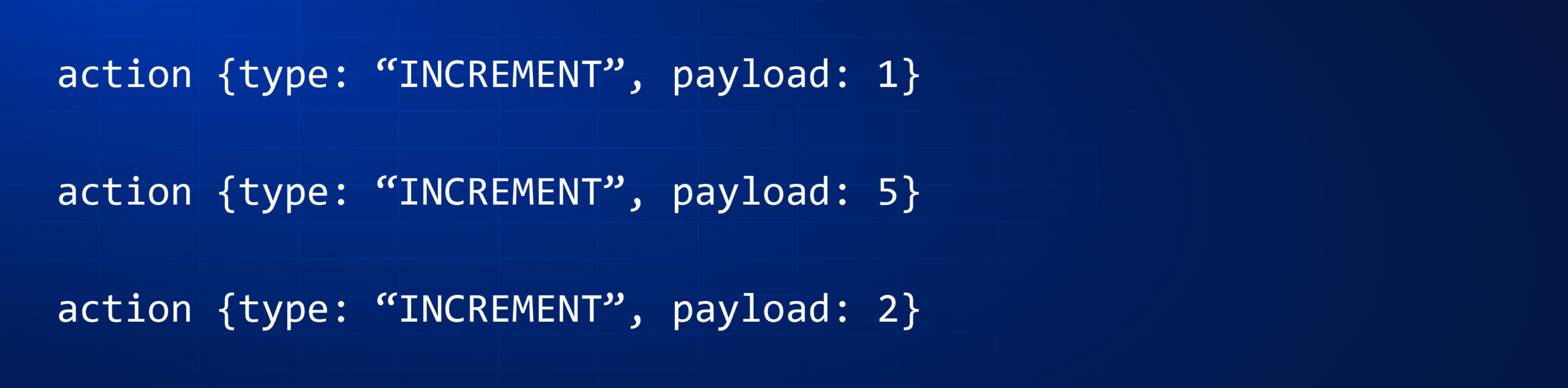
As you can see , we only get the actions logged to the console because the action is not forwarded to the reducer.
So this is how we can implement Redux Middleware.